by
kirupa | 29 July 2007
In the previous page,
you took the value converter you created and modified our data binding to use
it. The end result is that your application now works well! For the past few pages, we quickly ran through the details hoping to get our
data binding working. In this page, let's take a step back and look at the code
found in our value converter.
You copied and pasted much of the code that went
into our StringToBrush file. Let's look at each line
and see what it does:
- class
StringToBrush
:
IValueConverter
In this line, I define my StringToBrush class.
More importantly, I am implementing our
IValueConverter interface. In a nutshell, an
interface defines a contract. The contract specifies
details such as the number of methods and what
arguments each of your methods will take. The
interface itself contains no code beyond just
defining the methods and their arguments.
Our IValueConverter specifies the Convert and
ConvertBack methods. Like you saw in this tutorial,
with Visual Studio, once you simply specify an
interface to implement, the required methods are
created for you automatically.
- if
(value
!=
null)
- {
- string
input
=
value.ToString();
-
- if
(input.Length
!=
6)
- {
- throw
new
Exception("String
doesn't seem to be a valid RGB hex
color");
- }
-
- Color
newColor
=
(Color)ColorConverter.ConvertFromString("#"
+
input);
-
- SolidColorBrush
colorBrush
=
new
SolidColorBrush(newColor);
- return
colorBrush;
- }
- else
- {
- return
new
SolidColorBrush(Colors.White);
- }
In our Convert method, the first thing I check is
to make sure our value
variable is not null. The
value variable is important because it stores
the data that is passed in to our value converter.
If our value variable itself is null, that means
that no data was passed in.
To avoid having our
application spectacularly crash and burn, I return a
default SolidColorBrush in our
else statement in
the rare scenario when value does equal null.
All of the following code runs
when our value is not null (value != null) condition
holds.
- string
input
=
value.ToString();
With this line, I create a new object called
input, and it stores the string version of our value
object. Our value object is actually given to us as
a type object, and to avoid any issues associated with having a
generic object, I convert it to a string to better
deal with our data.
- if
(input.Length
!=
6)
- {
- throw
new
Exception("String
doesn't seem to be a valid RGB hex color");
- }
In this line, I make one more check to make sure
our input is valid. In this case, I check to make
sure that our provided value is six-digits long. If
the provided value is not six digits long, then I
throw an exception. I agree this is a naive way to
check whether an input is a good hex value
candidate, but for this tutorial, it does the trick.
- Color
newColor
=
(Color)ColorConverter.ConvertFromString("#"
+
input);
This line essentially fixes the shortcoming that
led to us creating our value converter. More
specifically, in this line, I create and initialize
our new Color objec using the
ColorConverter
object's ConvertFromString
method. The ConvertFromString method allows me to
take our 6-digit hex input and combine it with the
missing # character.
After this line executes, our newColor object
successfully took our incompatible hex code and with
the simple string manipulation, stores the color in
a form that WPF can understand.
- SolidColorBrush
colorBrush
=
new
SolidColorBrush(newColor);
- return
colorBrush;
We wrap up the work we started in the previous
line with these two lines. I take the new Color
object and create a SolidColorBrush out of it. The
reason is that our window's Background property
primarily deals with brushes, so it makes sense to
return our new color as a SolidColorBrush.
And with that, you are done with this value
converter tutorial.
Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
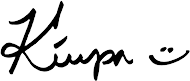
|