Loading a Random HTML Page Inline - Page 1
by
kirupa | 20 February 2011
Have questions? Discuss this HTML / JavaScript
tutorial with others on the forums.
Static is boring! One way of making your pages more dynamic is by
loading content that is a little different, a little
random each time. In this article, we forego
simpler, half-hearted approaches for doing this such
as by loading a random image, displaying some
elements in a different color, etc. Instead, you are
going to learn how to selectively load an entire HTML page!
For example, click (or keep clicking) on the display
random page button below to see a Good or Evil page display...randomly:
What you are seeing when you click the button is the
contents of the
good and
evil pages being loaded into the small region above this
paragraph and below the button.
By now, you are completely convinced as to the
awesomeness of doing this. Or, you may be wondering why you
may want ever want to do this in the first place.
The reason I originally explored this approach was for
displaying dynamic banner ads. I had an advertiser who
wished to show a different banner ad that consisted of a
different image, text link, URL, and alt tag. Because this
content went beyond just simple images pointing to the same
URL, I had to find another solution that wasn't image
specific. The solution was to first place each banner variation
inside a HTML page, and in the target page, selectively load the appropriate
banner HTML page instead.
The end result was what looked like a different banner
being displayed when someone visited the page! In this
article you will learn how to get something like this
working yourself.
Before we
dive into the code that describes how to load a HTML page,
let's describe the basic approach we are going to take.
To selectively load a different HTML page inside another
HTML page, we are going to be writing some JavaScript using
a heavy dose of Ajax. While I am not
going to go into great detail on Ajax in this article, just
know that it is a catch-all term for a series of web
technologies and techniques that help you to load content
on-demand seamlessly into an existing HTML page.
Besides the code, you need to specify a location where
the contents of the HTML file you wish to load will appear.
Once you put it all together, you have what is described in
the following diagram:
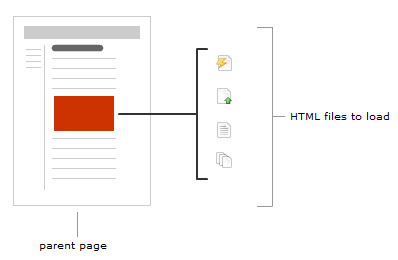
While this may all sound complicated, it's actually quite
straightforward once you start looking at each part in
greater detail!
To follow along with what I am explaining,
first make sure you have an HTML page created with a div
that you designate as the location you want to load your
external HTML page into. If you don't have an HTML page in
mind, feel free to just use the following:
- <html>
- <head>
- <title>Loading
an
External HTML
File</title>
- </head>
- <body>
- <div
id="contentArea">
- </div>
- </body>
- </html>
Notice that this is plain of an HTML page as you can get.
The only difference is that there is div with an id of
contentArea where I want
my external HTML pages to display in.
Speaking of external pages, you will need at least two
HTML pages that you can load. You can put pretty much
anything you want inside them, but just make sure those
pages are in the same domain as the page you are trying to
load them into. I will be referring to the two files in my
examples as
good.htm and evil.htm.
Once you
have your HTML pages setup, all that is left to do is to add
the code. Create a new JavaScript file called
inline.js and copy/paste the following code into
it:
- function
loadExternalHTMLPage()
{
- var
xmlhttp;
- var
pagesToDisplay
= ['good.htm',
'evil.htm'];
- if
(window.XMLHttpRequest)
{
- xmlhttp
=
new
XMLHttpRequest();
- }
else
{
- xmlhttp
=
new
ActiveXObject("Microsoft.XMLHTTP");
- }
- xmlhttp.onreadystatechange
=
function ()
{
- if
(xmlhttp.readyState
==
4
&&
xmlhttp.status
==
200)
{
- document.getElementById("contentArea").innerHTML
=
xmlhttp.responseText;
- }
- }
- var
randomnumber
=
Math.floor(Math.random()
*
pagesToDisplay.length);
- xmlhttp.open("GET",
pagesToDisplay[randomnumber],
true);
- xmlhttp.send();
- }
Before you save and close this file, replace good.htm and
evil.htm with the HTML pages you wish to load if you have
your own pages in mind. Note that the
path you specify should be relative to the HTML page you
wish to load your content into. The last thing to notice is
the second highlighted section where contentArea appears.
This is the ID of the div/element in the parent page that
you wish to load your HTML page into. Make sure to change it
if the div you are loading your HTML page into is
not called contentArea.
In your HTML file, make the following modifications so
that your JS file can be recognized:
- <html>
- <head>
- <title>Loading
an
External HTML
File</title>
- </head>
- <script
src="inline.js"
type="text/javascript"></script>
- <body
onload="loadExternalHTMLPage()">
- <div
id="contentArea">
- </div>
- </body>
- </html>
Right now, if you were to test your page, everything
should work. Each time you reload your document, one of the
two HTML pages you specified in your
inline.js file will have loaded.
w00t. In the
next page, we'll look at why this works.
Onwards to the
next
page!
|