by
kirupa | 6 June 2010
In the
previous page, we got about half way through the
tutorial when we looked at the code that is responsible for
displaying the file selection dialog. In this page, we'll
continue where we left off and start examining what happens
when a file has been selected.
We ended by looking at the event listener
that listens for the SELECT event and calls the selectFile
event handler when that event is overheard:
- function
selectFile(e:Event):void
- {
- file.addEventListener(Event.COMPLETE,
loadFile);
- file.load();
- }
The selectFile event handler does only two things. It
registers another event listener on our file object, and
this time, the event we are listening for is the COMPLETE
event. This event fires when what we are trying to load, the
file you selected earlier, has completely been loaded into
memory. This event listener will fire the loadFile event
handler.
While we have an event listener listening for an event
that will fire when we load our selected file, we haven't
actually told Flash to load anything yet. That is taken care
off in the second line with the
load function, also accessed through our
file object:
- file.load();
This line initiates loading the file, and when the file
has loaded, thanks to the event listener you declared one
line above, the loadFile
event handler will get called.
Let's look at that next:
- function
loadFile(e:Event):void
- {
- fileLoader
=
new Loader();
-
- fileLoader.contentLoaderInfo.addEventListener(Event.COMPLETE,
displayImage);
- fileLoader.loadBytes(file.data);
- }
In the first line, we initialize the fileLoad variable
that we talked about a while ago! The fileLoader object now
has access to the superpowers the Loader class contains. The
Loader class is responsible
for loading SWF files or images. For other types of files,
you have the URLLoader class
that you can use instead.
What we want to do is use our Loader object (fileLoader)
to load the image file you selected earlier and display it.
For this, you will need another event listener:
- fileLoader.contentLoaderInfo.addEventListener(Event.COMPLETE,
displayImage);
This time, our event listener is attached to our
fileLoader object's
contentLoaderInfo property.
This contentLoaderInfo property wraps the content you are
loading into a LoaderInfo object, and a LoaderInfo object is
the intermediate container your content needs to be in as it
makes its way from a Loader object (fileLoader) into
something that has been fully loaded. The diagram on
Adobe's AS3 Documentation page is helpful in making
sense of this.
The event listener attached to the
contentLoaderInfo property
will call the displayImage event handler when the COMPLETE
event has been fired. This event is fired when data loaded
into your fileLoader object has been fully loaded, and when
that happens, you tell
Flash to load the data in the following line:
- fileLoader.loadBytes(file.data);
The loadBytes function
takes the data returned by your
FileReference object file.
The
last thing that remains is to look at the displayImage event
handler that gets called when our image data is successfully
loaded by our Loader object,
fileLoader:
- function
displayImage(e:Event):void
- {
- addChild(fileLoader);
- }
This is probably the easiest line of code to explain in
this tutorial. Because we know that the image data has been
fully loaded, we can display it by just passing the
fileLoader object as the
argument to addChild. The
addChild function takes an object and puts it on the visual
tree for display, and fortunately, our fileLoader is one
object that actually has a visual component associated with
it - your image.
With your
loaded image displayed in your stage, we are now done with
this tutorial. Loading files from disk into a Flash
application is pretty straightforward. It can be distilled
into three steps as shown earlier. If you want to make this
a bit more generic so that you can load other file types
besides images, you will need to only make some minor
tweaks...hopefully.
If you are curious to see the full, working version of
this, download the source files from below:
Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
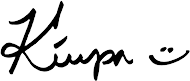
|