by Jesse Marangoni aka
TheCanadian | 30 May 2006
EventDispatcher is a class which provides a means of
dispatching events. After you’ve recovered from that
bombshell of an introduction sentence, let’s get more in
depth. EventDispatcher, like AsBroadcaster broadcasts an
event to a set of listeners which then receive that event
and call the function associated with it. If you have ever
used the Key class, you’ll be familiar with how you have to
add a listener to Key then set up events such as onKeyDown.
These events are in fact handled by AsBroadcaster but
EventDispatcher performs much the same function. If you ever
used components and such functions as addEventListener, you
will have definitely used this class before (even if not
knowing it) since EventDispatcher handles all v2 component
events. This tutorial will attempt to teach you the concepts
behind it and how to use it in your projects.
You can’t really place these two classes in direct
comparison since each has its strengths and weaknesses.
AsBroadcaster has one single collection of listeners which
receive every event broadcast. EventDispatcher has a group
of listeners for each event it broadcasts. Senocular paints
a nice picture:
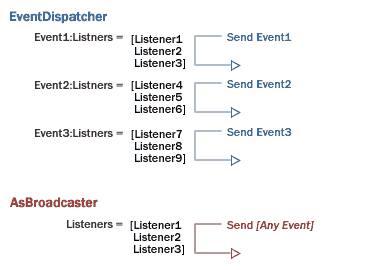
[ Comparison of
EventDispatcher and AsBroadcaster ]
It all boils down to which class is best for
each situation. EventDispatcher is good when you need
different listeners for different events. If you used
AsBroadcaster in this situation you would find yourself with
a bunch of listeners receiving events that they never use.
But if you need multiple listeners listening to the same
events it would be quite cumbersome to be adding those
listeners to listen for each event. This is where
AsBroadcaster shines.
For more info on AsBroadcaster and
listeners, see senoculars tutorial:
//www.kirupa.com/developer/actionscript/asbroadcaster.htm
One last thing to note about EventDispatcher is its ability
to have functions as a part of an event collection. With
AsBroadcaster this isn’t possible given that each listener
listens to every event but, since EventDispatcher is event
oriented, you can have functions which are called when the
event they are signed up to is broadcast.
Before we get to using it, you should have a brief
understanding of how a broadcasting object is initialized.
In its most basic sense, EventDispatcher is simply a
container for methods which are given to a broadcasting
instance when it is initialized. If we take a peek at its
static initialize method we can see how objects are set up
to begin broadcasting:
- static
function
initialize(object:Object):Void
{
- if
(_fEventDispatcher
==
undefined)
{
- _fEventDispatcher
=
new
EventDispatcher();
- }
- object.addEventListener
=
_fEventDispatcher.addEventListener;
- object.removeEventListener
=
_fEventDispatcher.removeEventListener;
- object.dispatchEvent
=
_fEventDispatcher.dispatchEvent;
- object.dispatchQueue
=
_fEventDispatcher.dispatchQueue;
- }
Calling that method just gives four methods
of an instance of EventDispatcher to the object passed to
the function. The object can now call these methods as if
they were its own – in its own scope. When broadcasting an
event, the broadcaster will loop through all listeners of
that event and call the function associated with it.
We have barely scratched the surface of how
this function works. Onwards to the
next page!
|