by
Granville Barnett | 15 September 2007
In the
previous page, you got a brief introduction to LINQ and
learned where to get the tools to get started. In this page,
let's continue on and create a simple LINQ application.
Let us dive in and take a look at LINQ in action by
performing a query on an in-memory collection. The goal of
this example is to get a core understanding of how a query
is constructed and what types of collections you can query:
- string[]
cities
= {
"London",
"Paris",
-
"Berlin", "Moscow",
"Dublin",
-
"Barcelona", "New York",
-
"Endinburgh", "Geneva",
-
"Amsterdam", "Madrid"
};
-
- IEnumerable<string>
query
= from
c in
cities
-
where c.StartsWith("M")
-
select c;
-
- foreach
(string
city
in query)
- {
- Console.WriteLine(city);
- }
If you are using Visual Studio 2008, create a new C#
Console Application and paste the above code inside your
Main method to see how it works.
In this snippet we use several standard operators to
construct a query which filters out all cities which start
with the letter ‘M’, however, of more significant importance
is the collection which we have queried.
We can query any in-memory collection which implements
IEnumerable, or
IEnumerable<T>. The example
previously shown calls the
GetEnumerator() on the query when the
foreach statement is
executed, subsequently a while
loop iterates over all items in the collection that satisfy
the query until the MoveNext()
method of the collection returns false.
If we run this example in debug mode, placing a
breakpoint on the same line as the
foreach statement, we can see that the compiler is
doing some extra work for us under the covers:
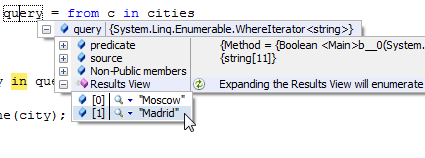
[ Calling a lambda expression ]
An implementation detail of the query given above is that
we are actually creating a lambda expression when the code
is compiled, this expression is used as a parameter for the
Where() extension method of
the cities collection.
Lambda expressions introduce a functional style of
programming into both the C# and VB.NET languages.
Constructing a lambda expression is simple using the new
generic Func<A0 , ... , A4, T>
type, which like all other examples which use LINQ-specific
features so far, is a part of the
System.Query namespace.
We will now go ahead and replicate the previous query
using lambda expressions:
- Func<string,
bool>
filter
= c
=> c.StartsWith("M");
- var
query
= cities.Where(filter);
-
- foreach
(string
city
in query)
- {
- Console.WriteLine(city);
- }
Here we define a lambda expression which takes a single
string parameter and returns a bool,
a lambda expression typically takes the following structure:
parameters => expression. Another thing to note is that we
call an extension method called
Where() on the cities collection which takes a
predicate as an argument, extension methods exist for
several common query operators including
Select,
OrderBy,
SelectAll, etc. For a full
reference see the
official LINQ project site.
To learn more about functional programming I would
strongly recommend you look at the
Haskell language. Anyway, we looked a few simple
examples in this page. In the next page, we'll look at more
involved examples involving SQL data.
Onwards to the
next
page.
|