by
kirupa | 3 August 2009
In the
previous page, you drew a circle and converted it into a
movie clip whose class name is ColorfulCircle. It might not
make a lot of sense why that is relevant, but you'll see in
this page.
What we are going to do next is
add some code that will cause the circle movie clip we just
created to appear as we move our mouse. To do this, go back
to your timeline. You should see just one layer with one
blank keyframe:
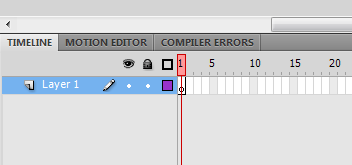
[ you will be adding some code to a blank keyframe ]
Right click on that keyframe and select Actions from the
menu that appears, select Actions. Once you have selected
Actions, the Actions window will appear. Copy and paste the
following code:
- function
duplicateCircle(e:MouseEvent)
{
-
- var
xPosition:Number=stage.mouseX;
- var
yPosition:Number=stage.mouseY;
- var
scaleFactor:Number=.5;
-
- var
circle:ColorfulCircle
=
new ColorfulCircle();
- circle.x=xPosition;
- circle.y=yPosition;
-
- circle.scaleX=scaleFactor;
- circle.scaleY=scaleFactor;
-
- this.addChild(circle);
- }
-
- function
startListening()
{
-
- stage.addEventListener(MouseEvent.MOUSE_MOVE,
duplicateCircle);
- }
- startListening();
Don't worry about what this code does just yet. In time,
I will explain in detail what each line of code does and how
it contributes to the final effect you will soon fully
create.
Getting back on track, if you test your application by
pressing Ctrl + Enter and moving your mouse around the Flash
player window, you will see something that looks like the
following:
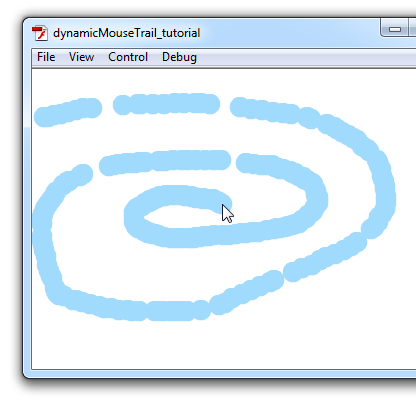
[ you have a mouse trail...literally ]
To look at the positve side first, you have a mouse
trail. The circle you drew earlier and made into a movieclip
is now being displayed at all the various locations your
mouse has been. What we need to do is make each circle
transition in and disappear once it is finished. Let's look
at that next.
As you recall, you defined a
class name called ColorfulCircle when you made your blue
circle into a movie clip. When you run your application, the
actual class is created for you by default. That allows you
to get away with not explicitly defining the class, but you
can't run away from that this time around.
In order to have your blue circles that appear at mouse
point transition in and disappear, you are going to have to
add some code that will live inside the ColorfulCircle
class. You will have to override the default behavior by
creating your own copy of the ColorfulCircle class that
Flash will read instead of creating one automatically.
This is pretty straightforward. From Flash, go to
File | New to display the New Document window. From this
window, select ActionScript file and press the OK button:
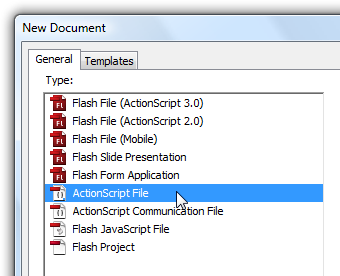
[ select the ActionScript file type from
New Document ]
Once you have clicked OK, the New Document window will
disappear, and your Flash drawing area will now be replaced
by what is essentially a large code editor.
Before we proceed any further, let's save this file. Go
to File | Save, navigate to the folder where your current
Flash project is, change the current default filename to
ColorfulCircle.as, and hit the Save button. A
ColorfulCircle.as file will now be created in the same
location as your dynamicMouseTrail.fla:
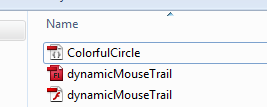
[ save your ColorfulCircle.as file in
the same location as your dynamicMouseTrail.fla ]
Great. We just created our ColorfulCircle.as file. Now,
its time for us to add some code to make all of this work.
With your ColorfulCircle.as file open in Flash, copy and
paste the following code into it:
- package
{
- import
flash.display.*;
- import
flash.events.*;
- import
flash.geom.ColorTransform;
-
- public
class
ColorfulCircle
extends
MovieClip
{
-
- var
speed:Number;
-
- public
function
ColorfulCircle()
{
- speed=.01+.02*Math.random();
- this.alpha
= .5;
- SetRandomColor();
- this.addEventListener(Event.ENTER_FRAME,
FadeCircleOut);
- }
-
- private
function
FadeCircleOut(e:Event)
{
- this.alpha-=.5*speed;
- this.scaleX+=5*speed;
- this.scaleY+=5*speed;
-
- if
(this.alpha<0)
{
- this.removeEventListener(Event.ENTER_FRAME,
FadeCircleOut);
- parent.removeChild(this);
- }
- }
-
- private
function
SetRandomColor()
{
- var
colorArray:Array
=
new
Array(0xFFFF33,
0xFFFFFF,
0x79DCF4,
0xFF3333,
0xFFCC33,
0x99CC33);
- var
randomColorID:Number
=
Math.floor(Math.random()*colorArray.length);
-
- var
myColor:ColorTransform
=
this.transform.colorTransform;
- myColor.color=colorArray[randomColorID];
-
- this.transform.colorTransform
=
myColor;
- }
- }
- }
After you have pasted all of the above code, run your
application again by pressing Ctrl + Enter. This time
around, notice that happens as you move your mouse around
the Flash Player window:
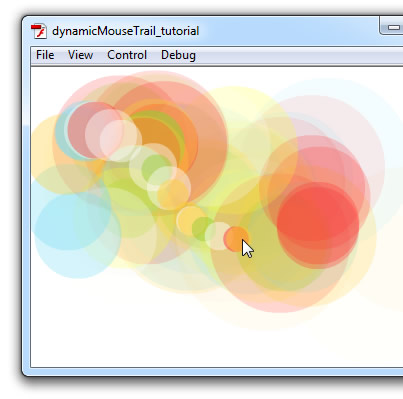
[ the circles....they are alive! ]
Wohoo! Your animation now works. Your animation working
is just (albeit big) hurdle for you to cross. The bigger,
more important one is knowing why the animation works the
way it does. So let's dive into our code and see what is
going on...on the
next page/a>!
Onwards to the
next page.
|