by
kirupa | 21 September 2009
In the
previous page, we began to look at the code that
causes your circles to have the effect that they do. In this
page, let's pick up where we left off.
- function
flyCircleIn(e:Event)
{
-
- radians
+=
Math.abs(speed/10);
-
- this.x
+=
Math.round(radius*Math.cos(radians));
- this.y
+=
Math.round(radius*Math.sin(radians));
-
- this.scaleX
+=
Math.abs(speed/10);
- this.scaleY
+=
Math.abs(speed/10);
- this.alpha
-=
Math.abs(speed/100);
-
- if
(this.alpha
<
0)
- {
- resetCircle();
- }
- }
The flyCircleIn function is responsible for creating the
movement that you see. If you recall, in the constructor,
you associated the Event.ENTER_FRAME event with this
flyCircleIn event handling function. This means that each
time your frame ticks this function gets called.
First, we increment our radians value by taking our speed
value and dividing it by 10:
- radians
+=
Math.abs(speed/10);
The radians value, like you saw earlier when I reset its
value to zero, determines where in the circular path your
circle currently is in. You'll see it used in the Circular
Motion code found in the next two lines:
- this.x
+=
Math.round(radius*Math.cos(radians));
- this.y
+=
Math.round(radius*Math.sin(radians));
The circular movement is calculated by using the Cosine
and Sine functions. Notice that I am passing our
radians value as an
argument to the Math.cos
and Math.sin functions,
and I am multiplying our Cosine
and Sine functions by the
radius value.
Now that we have the circular movement out of the way,
let's now look at the lines of code that animate the
circle's size and transparency:
- this.scaleX
+=
Math.abs(speed/10);
- this.scaleY
+=
Math.abs(speed/10);
- this.alpha
-=
Math.abs(speed/100);
These lines are very straightforward as well, and they
too involve the speed variable to determine how quickly the
resizing or fading out will occur.
When the circle fully fades out, we want to put an end to
the animation in its current state and reset everything to a
new set of properties:
- if
(this.alpha
< 0)
- {
- resetCircle();
- }
We accomplish that by checking when the circle becomes
fully transparent and calling the resetCircle function when
that happens. Speaking of which, let's look at the
resetCircle function next.
- function
resetCircle()
- {
- this.x
=
originalX;
- this.y
=
originalY;
-
- setProperties();
- }
The resetCircle function is called when our circles
become fully invisible. There are really only two things
this function does. First, it resets our circle'ss position
to what it was before it began its journey. Second, it calls
our setProperties function again where initial values are
set (or reset) for the next round of zooming, fading out,
and color changing.
The preceding
sections covered a lot of code, so let's use this moment to
quickly recap what our code is doing. Each of our circles is
like an independent life form with its own instance of
ColorfulCircle powering it. First, the constructor gets
called where the initial values of our circle are stored in
various variables and our enter frame event is associated
with our event handler.
At each tick of the frame, 24 per second if you keep the
default Flash CS4 value for frame rate, your circle
gradually becomes larger, moves in a circular path, and
becomes a bit less visible. Once your circle becomes fully
invisible, we reset the circle back to a good starting point
by calling the same function you called earlier for setting
the initial properties.
Even though we are calling the same function for setting
the properties, the properties that get set will probably
not be the same. There is a certain level of randomness that
is introduced where the speed, radius, and color variables
are set with the help of a Math.random call. This is nice
because it keeps your animation a bit more unique and lively
as each circle gets reset to a different variant of itself.
If you are interested in seeing my version of the code,
please feel free to download the source files below. The
colorfulExplosion.fla is what this tutorial used, and the
flyingCircles.fla represents the example animation from the
original page:
Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
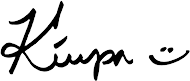
|