by kirupa |
24
December 2008
In the
previous page, you looked at our Name class and what
it contains. In this page, you will learn how to use
this class as part of an application.
Creating a class and populating it with properties and functions is just
one part of writing an application. An equally important part is to
create objects out of those classes. You've already done that, and the code for doing that can be found in
your FLA file where you pasted the following lines of code on a
keyframe:
- var author:Name
= new
Name();
- author.SetFirstName("Charles");
- author.SetLastName("Dickens");
-
- trace(author.GetFullName());
Let's look at the above lines of code in greater detail:
- var author:Name
= new
Name();
In the first line, we declare a variable whose name is author.
Beyond just giving it a name, we specify its type to be Name as wel. In case
you are wondering, the type Name is exactly the same Name class we
looked at a few moments ago.
Creating a variable is only the first half of what the above line
does. The second-half deals with creating your Name object. On the
right-hand side of the equal sign, you set the variable to an actual
Name object using the new keyword followed by
the Name constructor that you saw earlier
inside the name class.
This is where a constructor comes in handy. When you call a
constructor, you are essentially constructing a new object, and this is commonly
known also as instantiating an object. At the end of this first line, your author variable is now an
instance - a real manifestation of the class Name.
When you have an instance of a type, which is what we have with our
author variable, you have the ability to access the functions and any
variables from your type easily such as what I do in the following two
lines:
- author.SetFirstName("Charles");
- author.SetLastName("Dickens");
SetFirstName and SetLastName are both functions defined in your Name
class, but because the author variable is an instance of the name class,
you can call it directly from author itself.
Let's look at the final line of our code:
- trace(author.GetFullName());
In this line, I am tracing the value returned by the
GetFullName function from our author object. As you recall,
the GetFullName function returns a value of type string, and
this means that you can manipulate its output like you would
any other string-based data.
I am keeping things simple by just tracing the values
that you see. Next, let's look at this in a bit more
complicated light by creating many objects and visualizing
how they probably look.
To
help in explaining what is going on, the code you currently
have only creates a single object. Let's make things a bit
more interesting by creating many objects.
In the code you pasted in your Flash file's keyframe,
replace your current code with the following:
- //Book Author
- var
author:Name
= new
Name();
- author.SetFirstName("Charles");
- author.SetLastName("Dickens");
-
- trace(author.GetFullName());
-
- //Video Game Character
- var
game:Name
= new
Name();
- game.SetFirstName("Marcus");
- game.SetLastName("Fenix");
-
- trace(game.GetFullName());
-
- //TV Personality
- var
tv:Name
= new
Name();
- tv.SetFirstName("Mike");
- tv.SetLastName("Rowe");
-
- trace(tv.GetFullName());
-
- //Normal by day, Superhero by
night
- var
superHero:Name
= new
Name();
- superHero.SetFirstName("Bruce");
- superHero.SetLastName("Wayne");
-
- trace(superHero.GetFullName());
When you run your application now by pressing Ctrl +
Enter, the following is what you will see displayed in your
Output window:
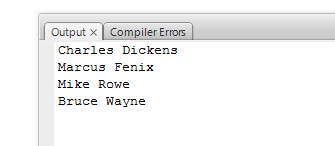
In the first page of this article, I showed you the
following diagram describing the relationship between
classes and objects:
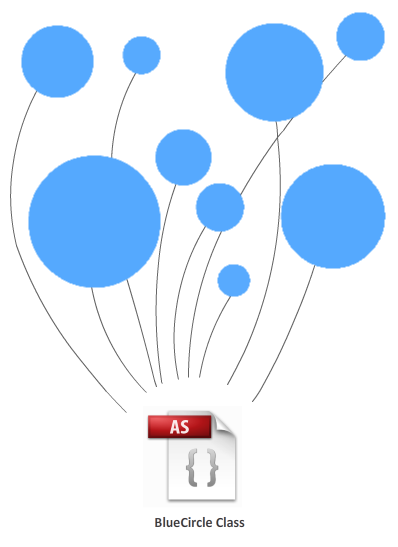
Let's reuse the idea from the above diagram and fill in
some missing details by visualizing the Name class and the
objects that it contains:
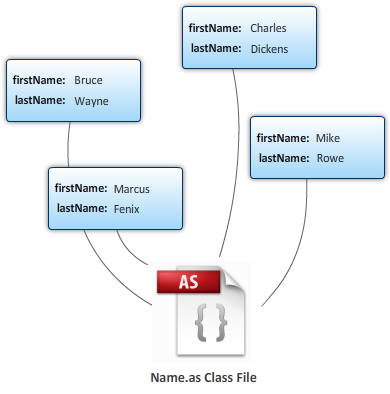
You have the four objects that we created based on the
Name class, and notice that each object stores the values
that are unique to it. In the above diagram, I have
populated the firstName
and lastName values.
Hopefully this article helped you to
get a better understanding of classes and their place in
modern applications. If you have done some minor
ActionScript 3 programming in Flash already, you probably
have used classes already. Any variable that you declare has
a type associated with it, and that type is basically a
class. For example, a common class you saw in this tutorial
is String, and there are dozens more built-in types that the
ActionScript language provides.
Fortunately, you are not limited to just using the
built-in types. As you saw in this article, creating your
own class is pretty straightforward. The Name class is
probably on the simpler side of the classes that you can
create, but even more complicated classes will follow a
similar pattern.
A great follow-up tutorial to read is
Classes and MovieClips where you learn how to associate
movie clips on your stage to classes, but otherwise, that's
it for this article! Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
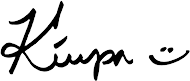
|