by
kirupa | 3 April 2006
In the previous page, I
finished explaining the part where the data was divided into
separate variables. I also provided a brief explanation as to
why we use so many variable names when dealing with strings in
the first place.
Now, we are ready to add the commas to separate our digits. For
example, a number such as 65536 should look like 65,536. The
trick lies in knowing just when to start adding a comma. There
are several ways to tackle this problem. The easy
way is to add commas by traversing your string in a
right-to-left direction.
Add the following colored code to do just that:
- dollarParser
=
function
()
{
- var
input:Number
=
1567.5644;
- var
inputString:String
=
input.toString();
- var
decimalIndex
=
inputString.indexOf('.');
- var
centString:String
=
inputString.substring(decimalIndex,
decimalIndex+3);
- trace(centString);
- var
dollarString:String
=
inputString.substring(0,
decimalIndex);
- //
- var
finalString:String
=
"$";
- var
count:Number
=
0;
- var
tempString:String
=
"";
- for (var
i:Number
= dollarString.length-1;
i>=0;
i--)
{
- count++;
- tempString +=
dollarString.charAt(i);
- if ((count%3
== 0)
&& (i
- 1
>= 0))
{
- tempString
+= ",";
- }
- }
- };
- dollarParser();
In the above code, in the for loop, I move backwards through our string:
- for (var
i:Number
= dollarString.length-1;
i>=0;
i--)
{
I start
at the end of our input by setting the count variable
i to equal the last character of our
string (dollarString.length-1) and I
keep looping until our counter, i, becomes less than 0. I
approaching zero is the same as you reaching the leftmost, first
character in our word list.
We want to add a comma after three characters. The easiest way
of doing so is to have a counter that increments and does
something when its value becomes a multiple of 3. You can do
that using the % (modulo) operator:
- if ((count%3
== 0)
&& (i
- 1
>= 0))
{
- tempString +=
",";
- }
Every time the count variable stores a number that is a multiple
of three, our tempString character gets a , comma added. Our
tempString variable, at the end of the loop, contains the
properly formatted dollars.
My if statement actually contains two conditions. The first
condition checks for multiples of threes. The second condition
checks to see if there is a number after your current position.
This ensures that, given a number such as 125, you do not end up
with a comma preceding it, for example - ,125.
Now, all we need to do is take our tempString data and insert it into our finalString variable that
currently only contains the $ sign. Add the following colored
lines of code to your existing code:
- dollarParser
=
function
()
{
- var
input:Number
=
1567.5644;
- var
inputString:String
=
input.toString();
- var
decimalIndex
=
inputString.indexOf('.');
- var
centString:String
=
inputString.substring(decimalIndex,
decimalIndex+3);
- trace(centString);
- var
dollarString:String
=
inputString.substring(0,
decimalIndex);
- //
- var
finalString:String
=
"$";
- var
count:Number
=
0;
- var
tempString:String
=
"";
- for
(var
i:Number
=
dollarString.length-1;
i>=0;
i--)
{
- count++;
- tempString
+=
dollarString.charAt(i);
- if
((count%3
==
0)
&&
(i
-
1
>=
0))
{
- tempString
+=
",";
- }
- }
- for (var
k:Number
= tempString.length;
k>=0;
k--)
{
- finalString +=
tempString.charAt(k);
- }
- };
- dollarParser();
The above code cycles through our tempString, takes each
character from it by using the charAt method, and adds it to the
end of our finalString variable.
Doesn't it seem inefficient to have two for loops that
essentially cycle through the characters in a string? Yes, it
is! The reason I use two for loops is because it is difficult to
gauge the length of our tempString string prior to the commas
being added. Therefore, adding values to our finalString after
tempString has been properly populated with numbers and commas
makes sense.
A better method would be to create an insert function that
inserts the commas into the appropriate location on the string,
but that is well beyond the scope of this tutorial.
There are more efficient ways, of course, but this tutorial is
primarily focused on Strings. I don't want to introduce any more
confusing conventions - at least not intentionally =)
At this point, tracing finalString will result in $1,234,567
being displayed. That's not bad, but we need to incorporate the
cents information we dealt with earlier! The final two lines of
code do that and finish up our re-creation of code I presented
at the beginning of this tutorial:
- dollarParser
=
function
()
{
- var
input:Number
=
1567.5644;
- var
inputString:String
=
input.toString();
- var
decimalIndex
=
inputString.indexOf('.');
- var
centString:String
=
inputString.substring(decimalIndex,
decimalIndex+3);
- trace(centString);
- var
dollarString:String
=
inputString.substring(0,
decimalIndex);
- //
- var
finalString:String
=
"$";
- var
count:Number
=
0;
- var
tempString:String
=
"";
- for
(var
i:Number
=
dollarString.length-1;
i>=0;
i--)
{
- count++;
- tempString
+=
dollarString.charAt(i);
- if
((count%3
==
0)
&&
(i
-
1
>=
0))
{
- tempString
+=
",";
- }
- }
- for
(var
k:Number
=
tempString.length;
k>=0;
k--)
{
- finalString
+=
tempString.charAt(k);
- }
- finalString +=
centString;
- trace(finalString);
- };
- dollarParser();
The following line sticks the data from our
centString variable to the end of our finalString
variable:
- finalString +=
centString;
When our output for finalString
complete, the trace command will output the following number:
$1,234,567.56
And with that, you now have a rudimentary decimal number
parser. It is not fully feature complete, and there are situations where you can
break the program easily. Try entering in non-numerical data such as letters and
see what happens, for example :-P
But, for the sake of understanding how
to use strings and the String class's methods in Flash, I hope this tutorial helped.
Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
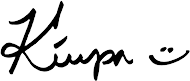
|