by
kirupa | 14 May 2008
In the
previous page,
you downloaded a sample project that contained the
animation that I've been describing for a while now.
To give you a better understanding of what goes on
behind the scenes, a bulk of the time in the
previous page was spent looking at XAML and
explaining keyframes, animations, and storyboards.
You will see in this page why that was done.
By now,
you may have forgotten what the original problem we
were trying to solve was. I know I certainly forgot.
You have an animation that currently plays at a
specified speed. What you want to do is change that
speed while the animation is running:
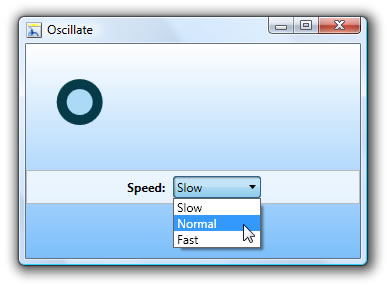
There are three speed values - Slow, Normal, and
Fast. When the Slow item is selected, our animation
will take longer to finish. When Normal is selected,
things move at a moderate speed. When Fast is
selected, your animation just flies through!
So, where is the speed property that we can
modify? Well, there is no value for speed. What you
do have are five values for duration as specified in
the keyframes...more specifically, our KeyTime
values in each of the SplineDoubleKeyFrames:
-
<Storyboard
x:Key="Oscillate">
-
<DoubleAnimationUsingKeyFrames
-
BeginTime="00:00:00"
-
RepeatBehavior="Forever"
-
Storyboard.TargetName="ellipse"
-
Storyboard.TargetProperty="(UIElement.RenderTransform).
(TransformGroup.Children)[3].(TranslateTransform.X)">
-
- <SplineDoubleKeyFrame
-
KeySpline="1,0,1,1"
-
KeyTime="00:00:00"
- Value="0"/>
- <SplineDoubleKeyFrame
-
KeySpline="0,1,1,1"
-
KeyTime="00:00:01"
- Value="-130"/>
- <SplineDoubleKeyFrame
-
KeySpline="1,0,1,1"
-
KeyTime="00:00:02"
- Value="0"/>
- <SplineDoubleKeyFrame
-
KeySpline="0,1,1,1"
-
KeyTime="00:00:03"
- Value="140"/>
- <SplineDoubleKeyFrame
-
KeySpline="1,0,1,1"
-
KeyTime="00:00:04"
- Value="0"/>
-
-
</DoubleAnimationUsingKeyFrames>
-
</Storyboard>
By decreasing the time between each keyframe, we
speed the animation up. That works because it now
takes less time for your circle to go from one edge
of the window to the other edge. Of course, by
increasing the time between each keyframe, we slow
the animation down. The justification is similar. It
takes your animation longer to do what it needs to
do.
What we are going to do is, each time the combo
box's value is changed, programmatically alter the
KeyTime values for each of our keyframes based on
what was selected. The next section will show you
how.
Finally, we get to write some code!
Like I mentioned earlier, we are going to alter the
KeyTime values in each of our keyframes when the
combo box's selection is changed. There are two
parts to this. The first part is finding out what
got selected in our combo box, and the second part
is changing the KeyTime values based on what was
selected.
If you look at the code for Window1.xaml.cs in
Visual Studio, you will recall that the ChangeSpeed
method is the event handler tied to our combo box's
SelectionChanged event. That means that each time
what you select inside the combo box changes, the
ChangeSpeed method gets called. That's exactly what
we want!
Inside your ChangeSpeed method, add the following
code:
- private
void
ChangeSpeed(object
sender,
SelectionChangedEventArgs
e)
- {
- double
incrementer
=
1;
-
- ComboBox
comboBox
=
sender
as
ComboBox;
- ComboBoxItem
selectedItem
=
comboBox.SelectedValue
as
ComboBoxItem;
-
- string
speed
=
selectedItem.Content.ToString();
-
- if
(speed
==
"Slow")
- {
- incrementer
=
2;
- }
- else
if
(speed
==
"Normal")
- {
- incrementer
=
1;
- }
- else
if
(speed
==
"Fast")
- {
- incrementer
= .5;
- }
- ModifyAnimation(incrementer);
- }
With
the above code, I am getting the selected value
(Slow, Normal, or Fast) and associating a number I
call the incrementer to each value. If Slow is
selected, my incrementer value is
2, for Normal it
is 1, and for
Fast it is .5.
The last thing
I do is call a ModifyAnimation method that takes our
incrementer value as its argument. Don't worry - you
haven't created that method yet. I am not going to
spend too much time on this section of code since it
deviates a bit from our stated goal of learning how
to modify animations created in XAML using C#.
Onwards to the
next page!
|