In Flash, you have the onMouseMove event that executes any code when your
mouse is moving. In some cases, executing code simply based on mouse movement
may not be enough. How would you like to learn how to determine the direction of
mouse movement so that you can execute different pieces of code depending on
which direction the mouse is moving?
For the ActionScript 3 version of this
code, please see the
following blog post.
In this short tutorial, I will explain just that. The code is based on
Macaroni Ted's (see
thread) implementation of mouse movement direction. Here is the code:
- checkX =
function (dx,
oldVal, newVal)
{
- if (oldVal<newVal)
{
- trace("moving
right");
- } else
if (oldVal>newVal)
{
- trace("moving
left");
- }
- return
newVal;
- };
- checkY =
function (dy,
oldVal, newVal)
{
- if (oldVal<newVal)
{
- trace("down");
- } else
if (oldVal>newVal)
{
- trace("up");
- }
- return
newVal;
- };
- this.watch("xdir",
checkX);
- this.watch("ydir",
checkY);
- this.onMouseMove
= function()
{
- xdir =
_xmouse;
- ydir =
_ymouse;
- };
Simply paste the above code onto a frame in Flash, and preview the animation
in Flash. Notice that the code traces the direction your mouse is moving!
Code Explanation
As always, here is an explanation of why the code does what it does.
- this.onMouseMove
= function()
{
Any code contained in the function referred by the onMouseMove event handler
will execute when the mouse cursor moves.
- xdir =
_xmouse;
- ydir =
_ymouse;
I create two new variables, xdir and ydir to store the x and y
position of our mouse. Since they are both contained within the onMouseMove
event handler, the values of xdir and ydir only change when the
mouse cursor is moved.
- this.watch("xdir",
checkX);
- this.watch("ydir",
checkY);
This brings us to the most interesting part of the tutorial, and to a topic
that has not been covered on this site at all: the watch function.
The watch function is pretty neat. What it does, is if a property or variable
it is interested in is changed, it calls on a callback function to do some
"stuff". I'm being intentionally vague, but you will be overwhelmed with details
shortly.
In our code, the variables our watch function is watching is xdir and
ydir variables. If you remember, they both store the x and y position of
your mouse cursor. When either of those variables change due to you moving the
mouse, the watch function is calls either the checkX or checkY
callback function depending on whether xdir or ydir changed.
The callback functions that the watch function calls on have to fit the
following format for most cases:
- callback(prop,
oldVal, newVal)
To recap, our watch function checks to
see whether the xdir or ydir variables have been changed. If any of these
variables did change, then it calls on either the checkX or checkY functions.
- checkX =
function (dx,
oldVal, newVal)
{
- if
(oldVal<newVal)
{
- trace("moving
right");
- }
else
if
(oldVal>newVal)
{
- trace("moving
left");
- }
- return
newVal;
- };
The above is our callback function for
checking movement in the x direction, and notice that it takes in the three
arguments I mentioned earlier.
When the checkX function is called
initially, oldVal is undefined and newVal contains the x position of your mouse
cursor. As you keep moving the mouse, you will notice that your new mouse
position replaces the old newVal, and that the old newVal becomes your new
oldVal.
To reduce the confusion of my above
paragraph, here is a series of trace actions that highlight what happens as the
mouse moves:
oldVal is: undefined and newVal is: 102
oldVal is: 102 and newVal is: 135
oldVal is: 135 and newVal is: 137
oldVal is: 137 and newVal is: 141
oldVal is: 141 and newVal is: 143
oldVal is: 143 and newVal is: 145
oldVal is: 145 and newVal is: 147
Notice how the initial value for oldVal
is undefined, and the values for newVal and oldVal alternated as I kept moving
the mouse. The reason oldVal and newVal alternate, is because of your return
statement:
- return newVal;
Because you return newVal, the watch
function's oldVal variable is replaced with the value from newVal.
- if (oldVal<newVal)
{
- trace("down");
- } else
if (oldVal>newVal)
{
- trace("up");
- }
This code is fairly straightforward.
You check to see if oldVal is less than newVal or if oldVal is greater than
newVal. oldVal is analogous to your old mouse position and newVal is analogous
to your new mouse position. I simply have a trace function that traces which
direction the mouse is moving, but you would probably replace it with code that
does something...useful!
For more information and gritty details
of the watch function, take a look at
Macromedia's LiveDoc article on it.
There are
several versions of the mouse direction detection script that I and others
created before settling on Marconi Ted's version, and you can find them all
here:
//www.kirupa.com/forum/showthread.php?t=186469
Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
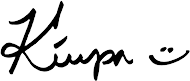