by
kadaj and kirupa | 19 January 2010
If you glance at a media player while it is playing back
mp3 files, you will see details such as the name of the song
that is currently playing, the artist, and other related
information:
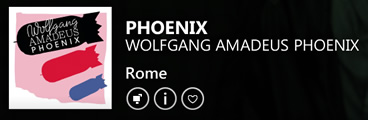
[ Rome Rome Rome Rome Rome Rome....
]
Have you ever wondered where the song details actually
come from? They come from the MP3 file itself. Today, a
standard MP3 file contains not only the data that makes up
your music, but it also contains metadata stored in the form
of ID3 tags.
It is these ID3 tags that store all of the information
about a song that you see, and in this article, you will
learn how to read these values in Flash.
When you
are working with sound in Flash, more than likely, you are
using the Sound class to load and play back the sound. Here
is a basic snippet of what code looks like to load an mp3
file and play it back once it has fully loaded:
- var
mySound:Sound;
-
- function
setup()
- {
- mySound
=
new Sound();
- mySound.load(new
URLRequest("07
- Rome.mp3"));
- mySound.addEventListener(Event.COMPLETE,
soundLoaded);
- }
- setup();
-
- function
soundLoaded(event:Event):void
- {
- mySound.play();
- }
Since this article isn't about sound, I am not going to
into great detail about how it works. There only two things
you need to note. The soundLoaded
event handler gets called when the sound file has loaded,
and our Sound object is declared globally and called
mySound.
One of
the properties the Sound class exposes is called
id3. As you can guess from
its name, it stores the metadata the MP3 sound file exposes.
To use the id3 property, you need to do two things. First,
you need ensure your mp3 file has an ID3 property. Second,
you need to access the property itself.
The best way to check if your loaded file
has an ID3 region is to use the Event.ID3 event on your
sound object. The non-grayed out lines show you how I set
that up:
- var
mySound:Sound;
-
-
function
setup()
- {
-
mySound
=
new
Sound();
-
mySound.load(new
URLRequest("07
- Rome.mp3"));
-
mySound.addEventListener(Event.COMPLETE,
soundLoaded);
- mySound.addEventListener(Event.ID3,
id3Loaded);
- }
- setup();
-
-
function
soundLoaded(event:Event):void
- {
-
mySound.play();
- }
-
- function
id3Loaded(event:Event):void
- {
-
- }
I set up the event listener on my sound object...aptly
called mySound...and associate the
Event.ID3 event with the
id3Loaded event handler. If my sound file has an ID3
region, this event will get fired and the id3Loaded function
will get called.
Note You may find that your
Event.ID3 event handler gets called more than once. Thanks
to lewi-p on the forums who did some investigation, it has
to do with the MP3 file having more than one ID3 tag
specified. You can read the
full thread here.
Now that you know your mp3 file
has some ID3 data, reading them is as easy as taking your
Sound object, mySound,
and accessing the id3
property:
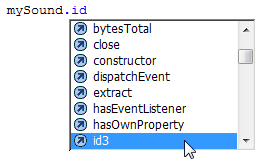
[ the id3 property lives directly on you Sound object ]
From this id3 property, you can get direct access to the
album name (album), genre (genre),
song name (songName), artist
(artist), track (track),
year (year), and comment (comment).
In my id3Loaded event handler, I have added some trace
statements that display the album information using the id3
properties that I listed in the previous sentence:
- function
id3Loaded(event:Event):void
- {
- trace("Album:
" +
mySound.id3.album);
- trace("Song:
" +
mySound.id3.songName);
- trace("Artist:
" +
mySound.id3.artist);
- trace("Track:
" +
mySound.id3.track);
- trace("Year:
" +
mySound.id3.year);
- trace("Comment:
" +
mySound.id3.comment);
- }
When I run my application, notice that the relevant
details are displayed in my Output window:
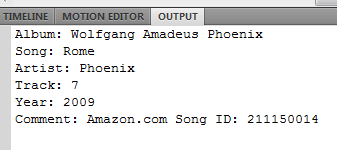
[ the song's ID3 information is now displayed ]
While I only displayed the six properties actually
defined, the Flash player actually exposes most (if not all)
of the ID3 tags that do not have properties associated with
them. You can view all of those properties in
Adobe's Documentation for ID3.
Hopefully you
found this short article helpful. As you can see, accessing
the ID3 values from an MP3 is greatly simplified because the
Sound class provides you with everything you need. You are
saved the hassle of having to read the various regions that
make up the MP3 file and parse out the relevant information
manually.
If you have any questions, please post them on the
kirupa.com Forums.
Cheers!
 |
kadaj
|
 |
kirupa
|
|